Začíname už za
# Part 08
miroslav.binas@tuke.sk / [**SMART**](https://kurzy.kpi.fei.tuke.sk/smart)
## Example: Torch App
Turn On
## What is Component?
> Component is small isolated, independent and reusable part for componsing the UI. ([React Native](https://reactjs.org/docs/components-and-props.html))
## Share Data Between the Components
## Relationships between Components
1. parent to child component
2. child to parent component
3. between siblings
4. sharing data between not related components
## Parent to Child
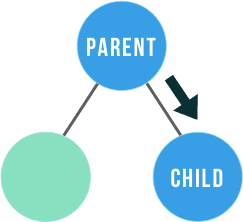
## Properties
## Component and it's Props
```javascript
function Hello(props){
return (
<Text>
Hello {props.name} {props.surname}!
</Text>
);
}
```
## Passing Props
```javascript
return (
<View style={styles.container}>
<Hello name="Sherlock"
surname="Holmes">
</Hello>
</View>
);
```
## Properties are Read-Only!
they are immutable!
## Pure Function
**All React components must act like pure functions with respect to their props.**
## Child to Parent
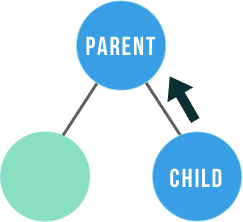
## Between Siblings
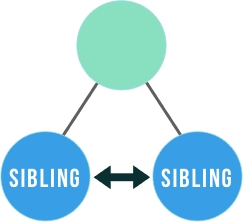
## Sharing data between not related components
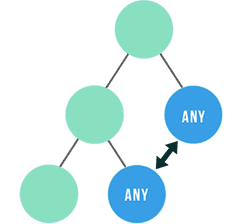

## Existing Approaches
* Global Variables
* Context
* Observer Pattern
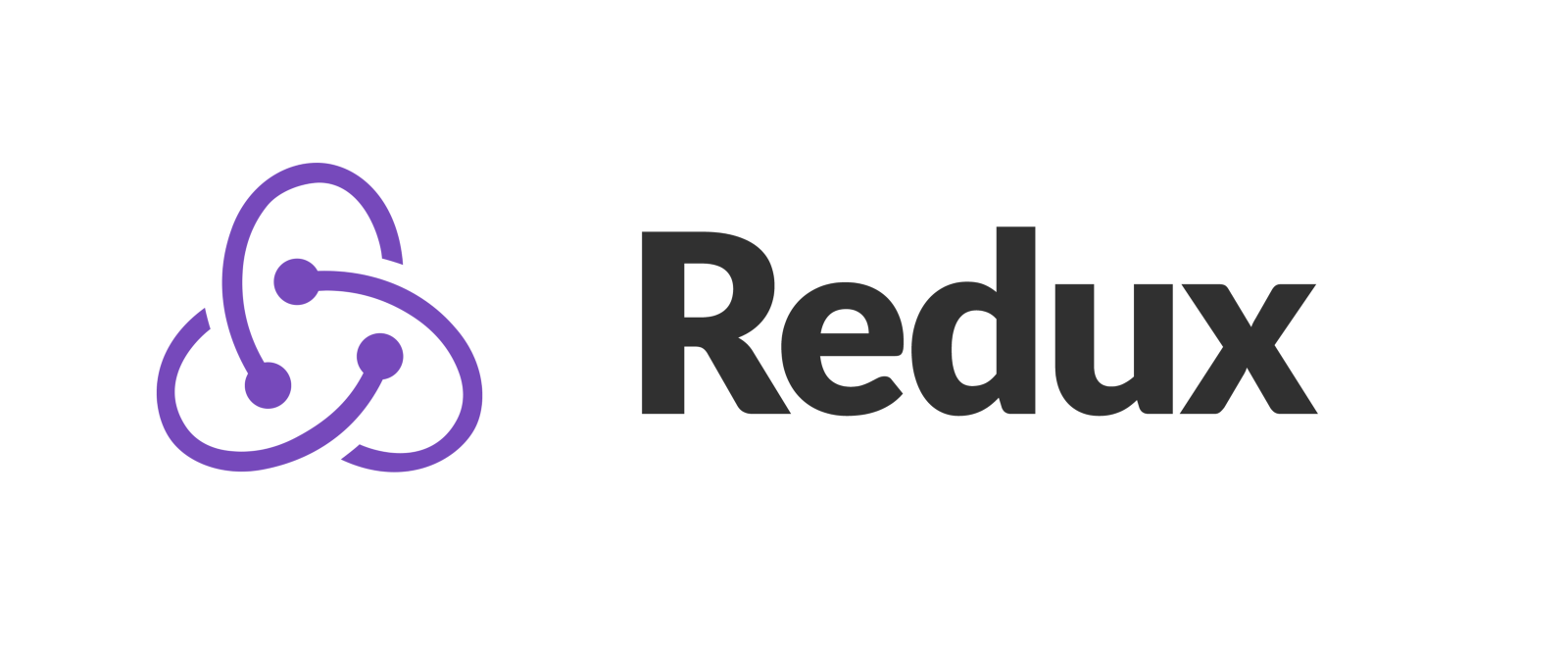
## Questions?