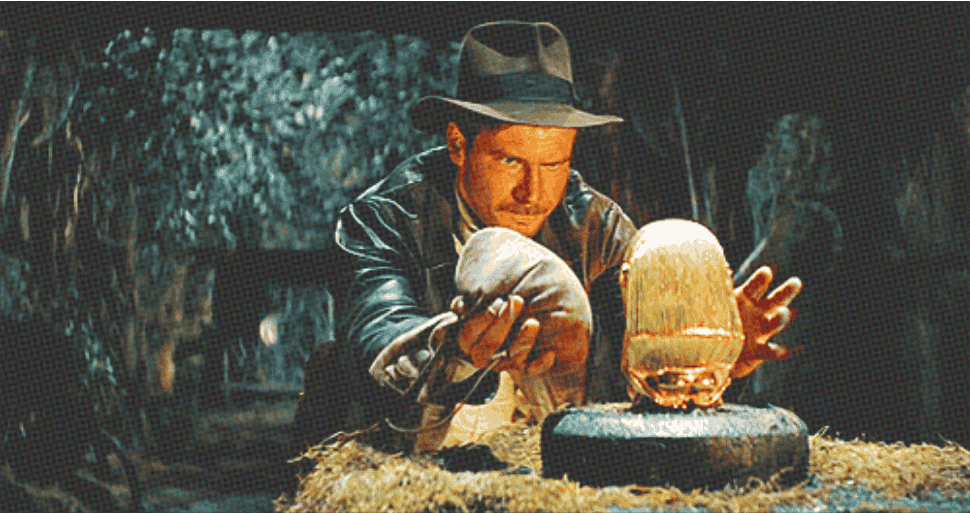
notes:
* https://aminoapps.com/c/movies-tv/page/blog/day-3-favorite-action-adventure/ewc3_up4d3LleL45bb1dNWq0RP7j2
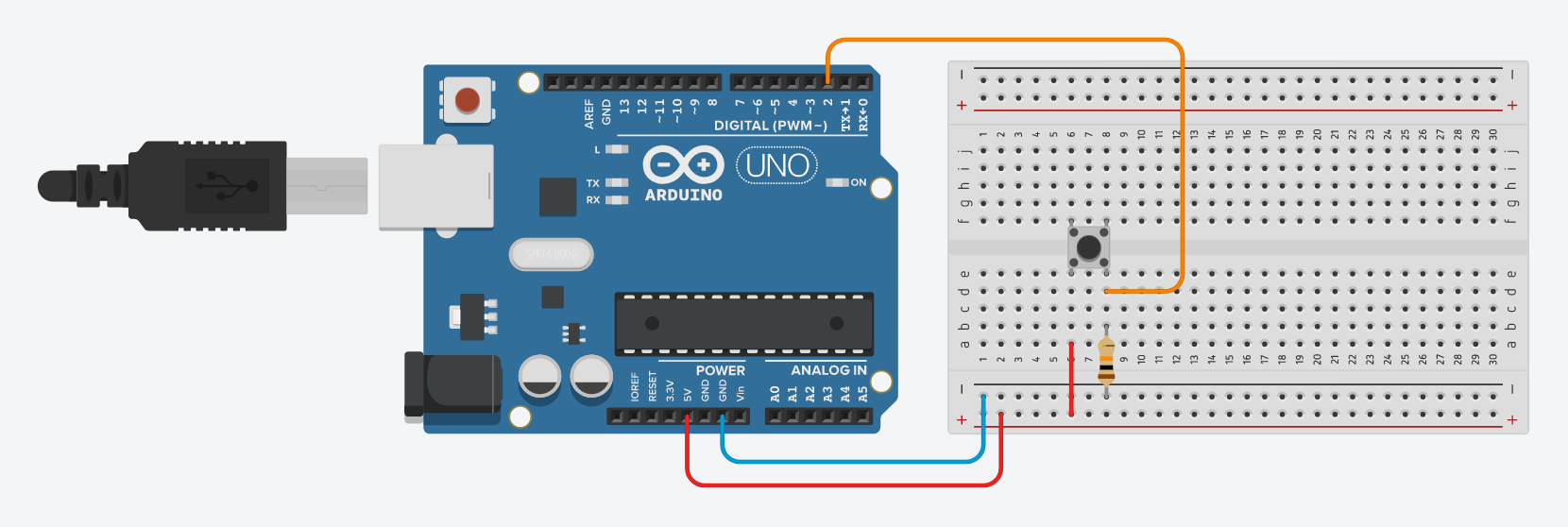
## Blocking Function Call
> **Blocking functions** prevent a program from doing anything else until that particular task has completed. ([Random Nerd Tutorials](https://randomnerdtutorials.com/why-you-shouldnt-always-use-the-arduino-delay-function/))
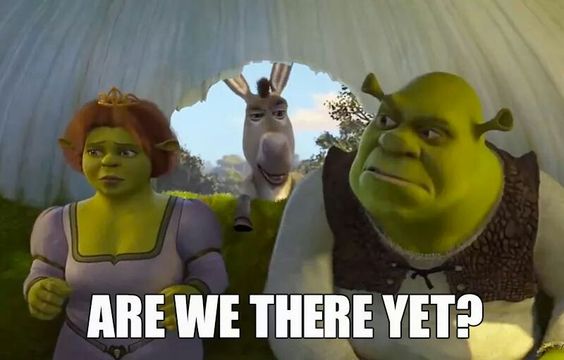
notes:
* https://dev.solita.fi/2020/06/08/introduction-to-spring-webflux.html
## Polling
> actively sampling the status of an external device by a client program as a synchronous activity
> ([Wikipedia](https://en.wikipedia.org/wiki/Polling_(computer_science))
## Interrupt
> signal to the processor emitted by hardware or software indicating an event that needs immediate attention
> ([Wikipedia](https://en.wikipedia.org/wiki/Interrupt))
## Interrupt Service Routine (ISR)
## When writing an _ISR_
* Keep it short!
* Don't use `delay()`!
* Don't do serial prints!
* Make variables shared with the main code `volatile`!
* Variables shared with main code may need to be protected by "_critical sections_"!
* Don't try to turn interrupts off or on!
Note:
* http://gammon.com.au/interrupts
## `attachInterrupt()`
* attaches interrupt to given pin
* syntax:
```cpp
attachInterrupt(
digitalPinToInterrupt(pin),
ISR,
mode
);
```
## Types of Interrupts
1. `LOW` - to trigger the interrupt whenever the pin is low
2. `CHANGE` - to trigger the interrupt whenever the pin changes value
3. `RISING` - to trigger when the pin goes from low to high
4. `FALLING` - for when the pin goes from high to low
## Reasons to use Interrupts
* To detect pin changes
* Watchdog timer
* Timer interrupts
* SPI data transfers
* I2C data transfers
* USART data transfers
* ADC conversions (analog to digital)
* EEPROM ready for use
* Flash memory ready
## Put Your Arduino to Sleep
```cpp
set_sleep_mode(SLEEP_MODE_PWR_DOWN);
sleep_mode();
```
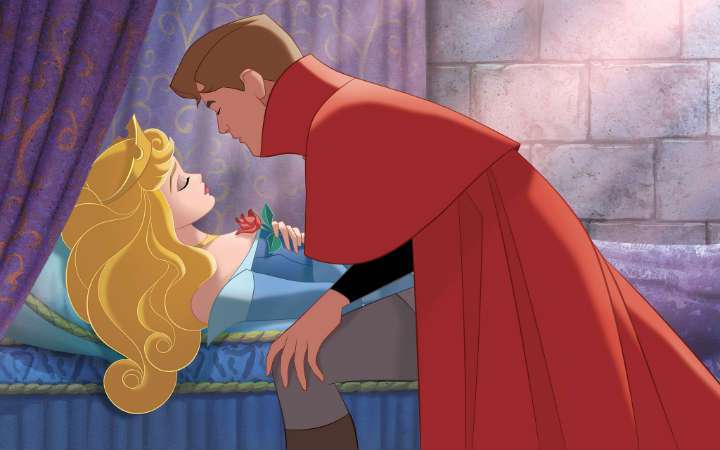
## Falling
```
HIGH
-------->-+
|
V
|
| LOW
+---------
```
## Rising
```
HIGH
+----------
|
|
^
LOW |
------->-+
```
[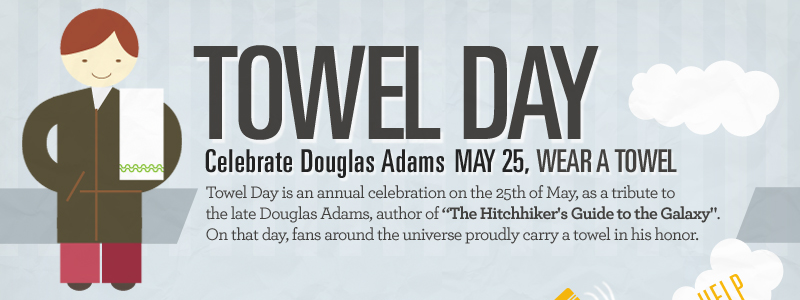](https://www.daysoftheyear.com/days/towel-day/)