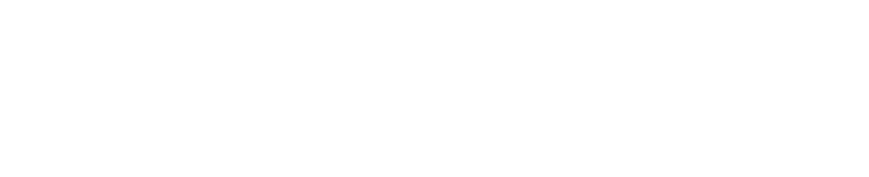
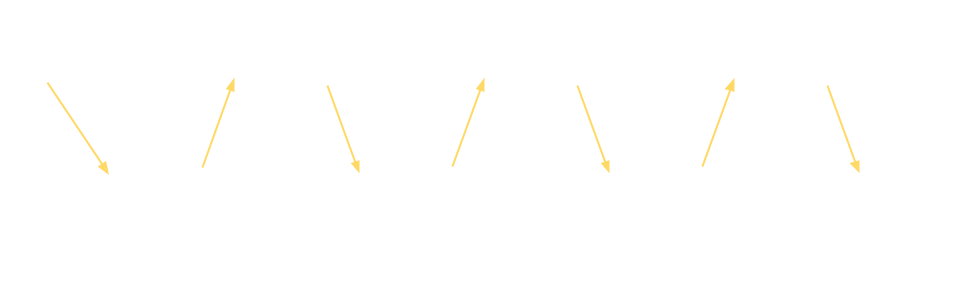

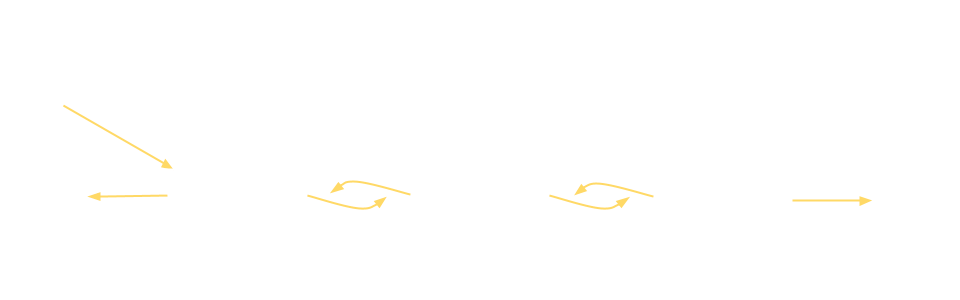
struct node {
int data;
struct node *next;
struct node *prev;
};
## [Circular Linked List](https://en.wikipedia.org/wiki/Linked_list#Circular_Linked_list)
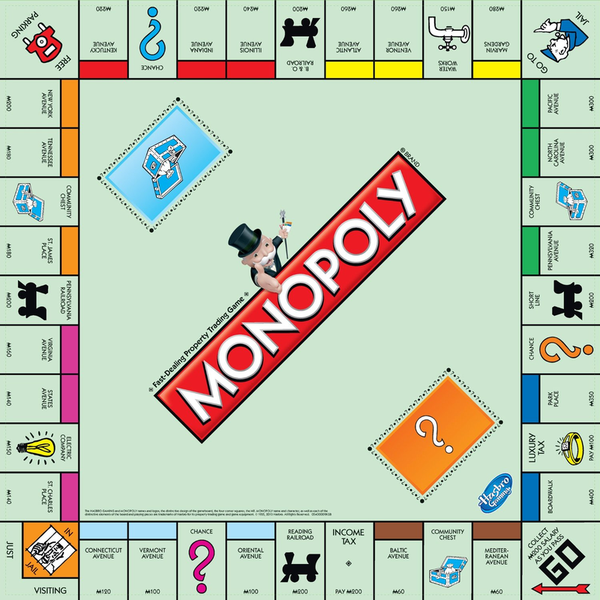
> **Tree** is a widely used abstract data type (ADT) that simulates a hierarchical tree structure, with a root value and subtrees of children with a parent node, represented as a set of linked nodes ([Wikipedia](https://en.wikipedia.org/wiki/Tree_(data_structure))
[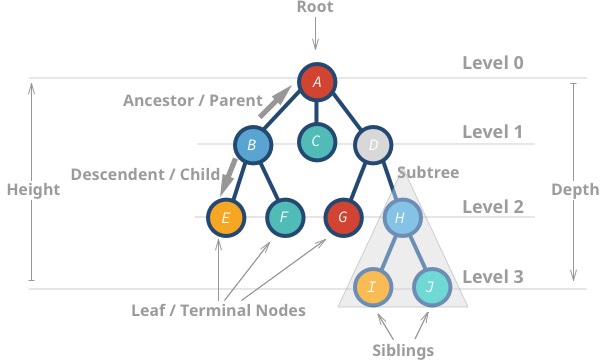](https://adrianmejia.com/data-structures-for-beginners-trees-binary-search-tree-tutorial/)
notes:
* https://adrianmejia.com/data-structures-for-beginners-trees-binary-search-tree-tutorial/
[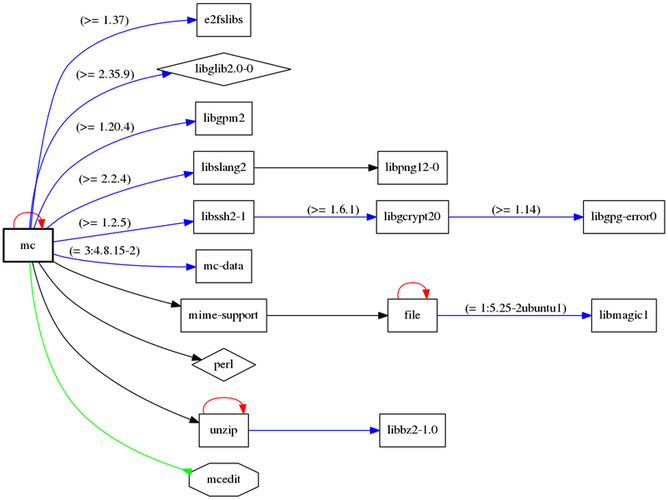](https://securitronlinux.com/bejiitaswrath/create-a-graph-of-package-dependencies-with-the-linux-command-line/)
notes:
* https://securitronlinux.com/bejiitaswrath/create-a-graph-of-package-dependencies-with-the-linux-command-line/
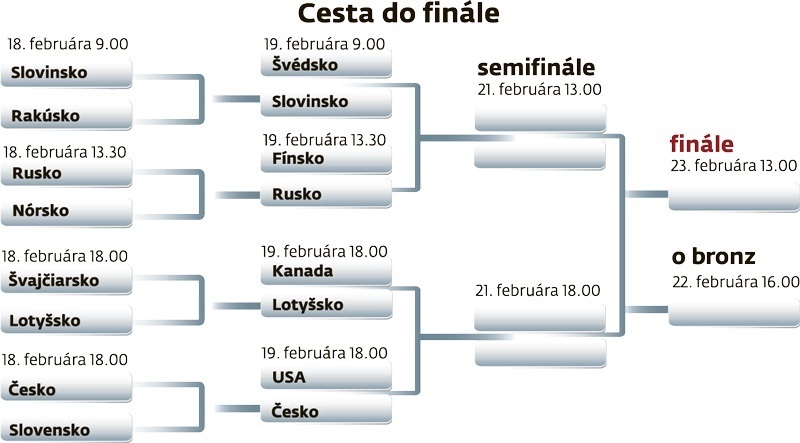
notes:
* https://sportnet.sme.sk/spravy/pozrite-si-stvrtfinalove-dvojice-v-soci-cechov-cakaju-americania/
prog-2024/
├── ps1/
│ ├── playfair.c
│ ├── playfair.h
│ ├── bmp.c
│ ├── bmp.h
│ ├── main.c
│ └── Makefile
└── README
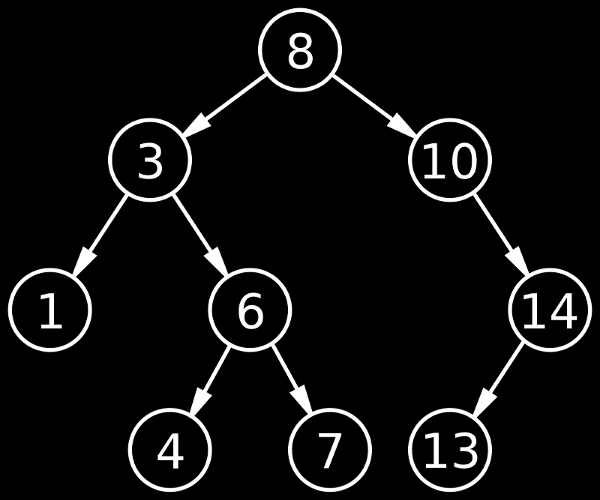
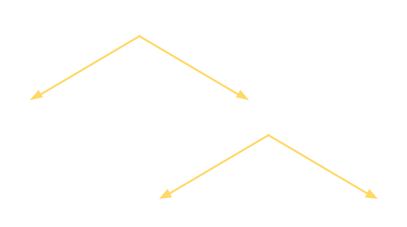
```cpp
struct node {
char* data;
struct node *yes;
struct node *no;
};
```
```cpp
struct node* create_node(
char* data
);
```
> A **union** is a special data type in C that allows to store different data types in the same memory location. You can define a union with many members, but only one member can contain a value at any given time. Unions provide an efficient way of using the same memory location for multiple-purpose. ([TutorialsPoint](https://www.tutorialspoint.com/cprogramming/c_unions.htm))
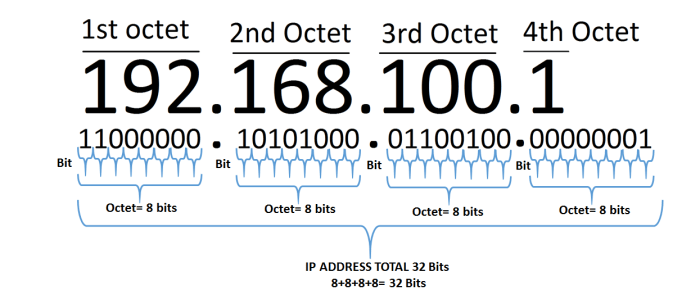
## ping
* dot decimal - `127.0.0.1`
* dot hexa - `0x7F.0x00.0x00.0x01`
* dot octal - `0177.0000.0000.0001`
* hexa number - `0x7F000001`
* decimal number - `2130706433`
* octal number - `017700000001`
```
ADDR | ADDR + 0 | ADDR + 1 | ADDR + 2 | ADDR + 3 |
+---------------+---------------+---------------+---------------+
| .as_number |
+---------------+---------------+---------------+---------------+
| .as_octets[0] | .as_octets[1] | .as_octets[2] | .as_octets[3] |
+---------------+---------------+---------------+---------------+
| .as_struct.d | .as_struct.c | .as_struct.b | .as_struct.a |
+---------------+---------------+---------------+---------------+
```
## Polymorphic Union
```
Connection
/ | \
Network USB VirtualConnection
```
```cpp
struct input_event {
enum event_type{
EventKeyPressed,
EventKeyReleased,
EventMousePressed,
EventMouseMoved,
EventMouseReleased
} type;
union {
unsigned int key_code;
struct{
int x;
int y;
unsigned int button_code;
};
};
};
```