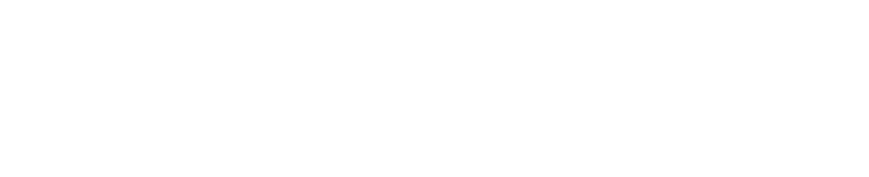
## CRUD
(**C**reate **R**etrieve **U**pdate **D**elete)
> In C programming, file is a place on your physical disk where information is stored. ([www.programiz.com](https://www.programiz.com/c-programming/c-file-input-output))

struct node {
char data[5];
struct node* next;
};
> Linked list is a data structure consisting of a group of nodes which together represent a sequence. ([Wikipedia](http://en.wikipedia.org/wiki/Linked_list))
## CRUD
- **C**reate
- **R**etrieve
- **U**pdate
- **D**elete
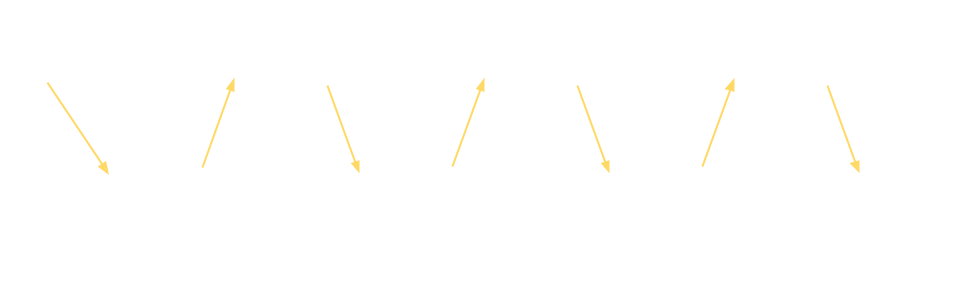
struct node {
int data;
struct node* next;
};
```cpp
void traverse(
struct node* head
);
```
```cpp
void append(
struct node** head,
int data
);
```
```cpp
struct node* pop(
struct node** head
);
```
```cpp
void insert(
struct node** head,
size_t index,
int data
);
```
```cpp
struct node* search(
struct node* head,
int needle
);
```
```cpp
struct node* delete(
struct node** head,
int needle
);
```
```cpp
void update(
struct node** head,
struct node* old,
struct node* new
);
```
```cpp
struct container {
struct node* head;
size_t size;
};
```
## Conclusion
* single linked lists
* other types of linked lists
* other data structures
* trees
* hash maps
* comming soon...