## Previously you Have Seen
```cpp
struct person {
char name[10];
char surname[20];
int age; // <-- WTF?
char sex; // M, F
};
```
## Date and Time Representation
## Current Time
now
Date and Time in a Structure
## Macros
```cpp
#define SUNDAY 0
#define MONDAY 1
#define TUESDAY 2
#define WEDNESDAY 3
#define THURSDAY 4
#define FRIDAY 5
#define SATURDAY 6
```
## Enumerated Type
> is a data type consisting of a set of named values called **elements** (or members/enumerals/enumerators) of the type
> ([Wikipedia](https://en.wikipedia.org/wiki/Enumerated_type))
```cpp
enum days {
SUNDAY,
MONDAY,
TUESDAY,
WEDNESDAY,
THURSDAY,
FRIDAY,
SATURDAY
};
```
```cpp
enum days {
SUNDAY, // 0
MONDAY, // 1
TUESDAY, // 2
WEDNESDAY, // 3
THURSDAY, // 4
FRIDAY, // 5
SATURDAY // 6
};
```
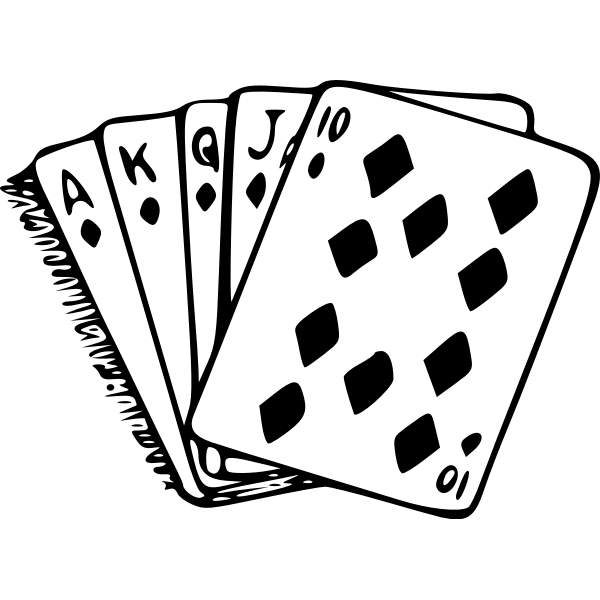
notes:
* https://freesvg.org/royal-flush
```cpp
enum suit {
CLUB,
DIAMONDS,
HEARTS,
SPADES
};
```
## Boolean Type
```cpp
enum boolean { false, true };
```
## Logging Levels
```cpp
enum loglevel {
DEBUG = 10,
INFO = 20,
WARNING = 30,
ERROR = 40,
CRITICAL = 50
};
```
## Overflow
## Integer Overflow
> occurs when an arithmetic operation attempts to create a numeric value that is outside of the range that can be represented with a given number of bits