## Week 04
miroslav.binas@tuke.sk / [**IoT1**](http://kpi.pages.kpi.fei.tuke.sk/iot1)
#### http://kpi.pages.kpi.fei.tuke.sk/iot1
#### https://mattermost.kpi.fei.tuke.sk/iot1
[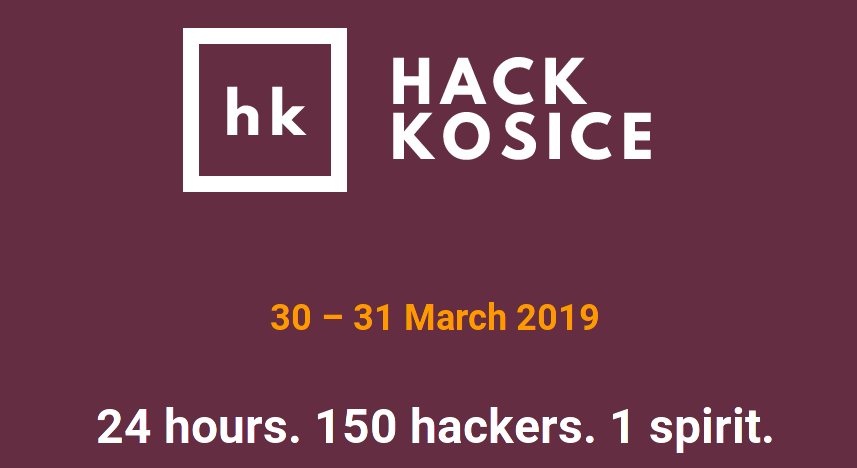](https://hackkosice.com/)
[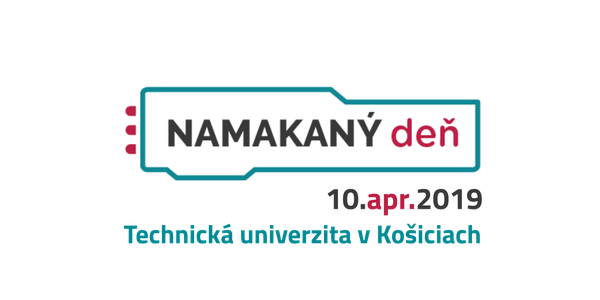](https://www.namakanyden.sk/)
## Internal Interrupts (Timers)
* run ISR at given time
* depends on frequency (ATmega328P uses external _16MHz_ oscilator)
## Arduino Timers
* `TIMER0` - 8b, used in time functions `delay()`, `millis()` and `micros()`
* `TIMER1` - 16b, used in `Servo.h`
* `TIMER2` - 8b, used in tone generator (`tone()` function)
all timers are used in function `analogWrite()` - each for different pins
## Types of Timer Interrupts
* **Compare Match Interrupt** - Interrupt occurs, when given value is reached.
* **Overflow Interrupt** - Each timer uses internal counter based on the used register. When the register overflows, interrupt occurs.
* **Input Capture Interrupt** - When expected event occurs on given pin (raising, falling or change).
## Timer Features
| Timer | Size | Range | Possible Interrupts |
|:--------:|:---------:|:---------:|:--------------------|
| `TIMER0` | _8b_ | _0-255_ | Compare Match, Overflow |
| `TIMER1` | _16b_ | _0-65535_ | Compare Match, Overflow, Input Capture |
| `TIMER2` | _8b_ | _0-255_ | Compare Match, Overflow |
## Watchdog Timer
* if device enters to error state, _Watchdog Timer_ is responsible to restart it after period of time
## TimerOne
* provides easy API for timers
* hides the low-level access provided by AVR libraries
* works with `TIMER1` only
## TimerOne API
* `Timer1.initialize(micros)` - initialization (must be called first), `micros` defines timer's period
* `Timer1.attachInterrupt(function)` - after period of time is executed given _ISR_
* `Timer1.detachInterrupt()` - detach interupt
* `Timer1.start()` - start timer with new period
* `Timer1.stop()` - stop the timer
* `Timer1.restart()` - restart the timer from start of new period
## Sleep Mode
* special mode of microcontroller
* low power mode (depends of what was turned off)
* state of microcontroller remains in it's memory
## Sleep Mode Illustrations
* common devices provides sleep mode too
* dvd player, tv, set-top box
* when turned off by remote
## Sleep Mode and Interrupts
* to wake up microcontroller from sleep mode, you can
* use interrupts
* use reset signal (which is interrupt too)
## Putting Arduino to Sleep
* include `avr/sleep.h`
* `set_sleep_mode(mode)` - sets the sleep mode, which can be one of `SLEEP_MODE_IDLE`, `SLEEP_MODE_ADC`, `SLEEP_MODE_PWR_SAVE`, `SLEEP_MODE_STANDBY` or `SLEEP_MODE_PWR_DOWN`
* `sleep_mode()` - enables sleep mode
## Arduino Sleep Modes
available in `sleep.h`:
* _Idle_ (`SLEEP_MODE_IDLE`)
* _ADC Noise Reduction_ (`SLEEP_MODE_ADC`)
* _Power-save_ (`SLEEP_MODE_PWR_SAVE`)
* _Standby_ (`SLEEP_MODE_STANDBY`)
* _Power-down_ (`SLEEP_MODE_PWR_DOWN`)
## Sleep Mode Example
```c
void setup(){
pinMode(LED_BUILTIN, OUTPUT);
set_sleep_mode(SLEEP_MODE_PWR_DOWN);
}
void loop(){
digitalWrite(LED_BUILTIN, HIGH);
delay(500);
sleep_mode();
digitalWrite(LED_BUILTIN, LOW);
delay(500);
}
```

(**http://bit.ly/2u1sPBh**)